include("dotnetindex.php") ?> |
|
Quick demo
I’m going to give a quick ASP.Net demo to show two of its best features.
I’m going to use two files. The first is almost entirely HTML. The
only extras are some ASP directives at the top, linking the page to
a C# file, the use of the asp: namespace for the form controls, and
the Runat=”Server” attribute on several of the controls.
View
the .aspx source
The second file is simply a C# class. It inherits from the Page
class, and declares as variables the HTML controls in the web page.
The code then treats those controls as if they lived on a Windows
form running locally. The button has a click event, which sets the
text of the label. OK, you could do this in 2 seconds using client-side
JavaScript, but I’m sure you can see that where you can do a simple
thing, you can also do something more complex using the same technique.
View
the C# source
When I run the page, I can click the button and see that the text
of the label changes. Of course what I’m actually doing is submitting
a form, and having a new version of the page returned to the browser.
Note that it’s pure HTML and runs on any browser. It’s still quite
possible to run into problems where stuff works on IE but not on other
browsers, but it’s not inherent to ASP.Net.
Try
the example
One last thing: if you click refresh you’ll notice that the label
text stays the same. This is the automatic page state preservation,
and can save a lot of time. It’s done by means of a hidden form variable
called Viewstate. Fortunately you have a lot of programmatic control
over viewstate and what happens when a page is submitted, so there
is no problem with disabling or restricting this feature when necessary.
There's a little more about ASP.Net in the Visual Studio section
below.
Windows Forms
Just as ASP.Net provides a library for web applications, Windows
Forms is a library for Windows applications. You saw a little of this
in the first C# demo I gave. Windows Forms wraps the Win32 API. It
makes it easy to assemble GUI Windows applications, using visual form
designers familiar to Visual Basic or Borland Delphi users. It’s actually
much nicer to use than VB, because the code generation process is
more transparent. You can do what you can do in Delphi but not in
earlier VB versions, which is to edit the code and have the designer
update itself, or vice versa. It’s also a lot easier to create custom
components.
Having said that, there are a few problems with Windows Forms. The
library does not implement a model – view – controller architecture,
which probably makes it easier for ex-VB coders, but less satisfactory
for some advanced programmers. There is a 3rd party solution for this
in the pipeline. Another issue is that the first release of Windows
Forms is somewhat buggy, with serious memory leaks in some areas.
I expect these will be mostly fixed in the coming 1.1 update. Third,
while performance of Windows Forms is reasonable, the just-in-time
compilation means slow load times, and in general Windows Forms applications
are fairly resource hungry. Fourth, the .Net runtime requirement is
prohibitive for anyone deploying applications over the web, at least
to dial-up users. Some developers are waiting for Microsoft to find
a way to distribute the runtime more widely. My own hunch is that
Internet Explorer 7.0, whenever it comes, will be the vehicle for
this.
There’s also the matter of disposing of unmanaged resources. In
the .Net Framework, the garbage collector looks after reclaiming memory,
but its timing is indeterminate. That makes it dangerous to put cleanup
code in the destructor, if you want to be sure that it runs as soon
as you’ve finished with the object. The solution to this is an interface
called IDisposable, which is implemented by classes that use unmanaged
resources like fonts, database connections, windows GUI resources
and so on. You call the Dispose method when you have finished with
the object, and this frees the resources. Unfortunately, properly
implementing IDisposable turns out to be a complex topic. It isn’t
only a problem for Windows Forms applications, but it is most significant
there. This is one of the least appealing features of the .Net Framework,
and while probably unavoidable, it’s important to be aware of it before
diving into a .Net project.
Getting back to the good stuff, Microsoft has implemented Windows
Forms on Windows CE as well as Win32. This makes it an excellent programming
tool for the Pocket PC. The .Net Framework is easier to use than J2ME.
Again, the snag here is that the runtime is not widely deployed: how
could it be, when the Compact Framework, as it is called, is actually
still in beta. However, I regard this as a promising area for .Net,
programming rich client application on devices, especially when a
wireless connection enables access to XML web services.
Visual Studio .Net
Visual Studio .Net is Microsoft’s development tool for .Net. It’s
pretty nice to work with, although Microsoft slipped up by not providing
any refactoring tools. It’s highly customizable, online help is well
integrated, the code completion is the best I’ve seen. One of its
best features is that building web pages is similar to building windows
applications. Here’s a quick demo. It’s a shopping list application,
and it always used to be used by IBM as a tutorial application for
its VisualAge tools. First, the Windows Forms version:
Now, to create this I really only wrote one line of code:
this.listBox1.Items.Add(this.textBox1.Text);
The application is assembled in true Visual Basic style (although
it's C#), drag and drop components from a palette, set properties,
double-click the button to open an event handler. Now a look at the
web version. FIrst, start a new Web application and drag components
to a web form:
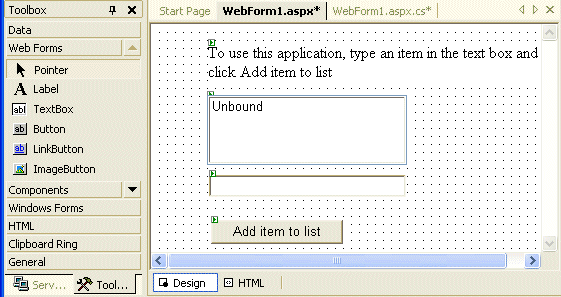
Next, double-click the button and add some code - the same code I
used for the Windows version:
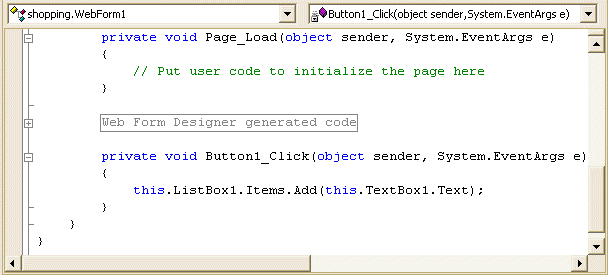
Finally, run the application. I'm using an open-source web browser:
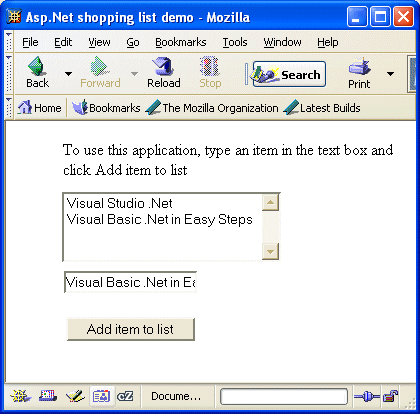
The whole process is very similar to that for a Windows application.
You
can try the application yourself here.
There’s another aspect to this. Say for example you are designing
a new business application to be used within your organisation. Should
it be a Windows application or browser-based? It’s a hard decision,
with the browser option compelling for broad reach and accessibility,
while the Windows option is good for taking advantage of local processing
power, displaying charts and graphs, interacting with Microsoft Office,
and so on. I reckon a great solution to this is to plan for both,
implementing the functionality of the application in libraries that
can be called either from a Web application or from a Windows application.
Because ASP.Net code is simply another .Net assembly, it’s easy to
do this.
Next
|